Mathematische Gleichungen in Python?
Hey,
kann mir jemand kurz helfen. Ich verstehe net ganz wie ich diese Gleichung in ein Python Script übertrage.
Danke im Vorraus 👍
3 Antworten
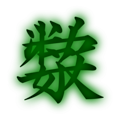
Was ist hier mit „in ein Python-Script übertragen“ gemeint? Was genau möchtest du denn haben? Vermutlich geht es dir um eine Funktion, welche Werte für o, v, c entgegennimmt und daraus die b-Werte berechnet, oder? Dann geht das beispielsweise so...
def berechne_b(o, v, c):
from math import sqrt
x = o/(4*c) - v/(2*c*c)
y = sqrt(x*x - v/c)
return(x - y, x + y)
... für die eine Gleichung, und so...
def berechne_b(o, v, c):
from math import sqrt
x = o/(2*c) - v/(c*c)
y = sqrt(x * x * v/c)
return(x - y, x + y)
... für die andere Gleichung.
------------------------
Beispiel:
def berechne_b(o, v, c):
from math import sqrt
x = o/(4*c) - v/(2*c*c)
y = sqrt(x*x - v/c)
return(x - y, x + y)
def berechne_b2(o, v, c):
from math import sqrt
x = o/(2*c) - v/(c*c)
y = sqrt(x * x * v/c)
return(x - y, x + y)
o = 20
v = 1
c = 1
print(f"Für o = {o}, v = {v}, c = {c} erhält man mit der einen Gleichung...")
b = berechne_b(o, v, c)
print(f"b[0] = {b[0]} und b[1] = {b[1]}")
print("... und mit der anderen Gleichung...")
b2 = berechne_b2(o, v, c)
print(f"b[0] = {b2[0]} und b[1] = {b2[1]}")
Output zum Beispiel:
Für o = 20, v = 1, c = 1 erhält man mit der einen Gleichung...
b[0] = 0.1125178063039387 und b[1] = 8.887482193696062
... und mit der anderen Gleichung...
b[0] = 0.0 und b[1] = 18.0
============
Oder möchtest du die Gleichung symbolisch zur Verfügung haben, um gegebenenfalls Umformungen, etc. bei dieser durchführen zu können? Das geht beispielsweise mit dem SymPy-Modul.
Für den einen Fall dann...
from sympy import symbols, Eq, sqrt
b, o, v, c = symbols('b o v c')
Gleichung1 = Eq(b, o/(4*c) - v/(2 * c**2) - sqrt((o/(4*c) - v/(2 * c**2))**2 - v/c))
Gleichung2 = Eq(b, o/(4*c) - v/(2 * c**2) + sqrt((o/(4*c) - v/(2 * c**2))**2 - v/c))
Und für den anderen Fall dann...
from sympy import symbols, Eq, sqrt
b, o, v, c = symbols('b o v c')
Gleichung1 = Eq(b, o/(2*c) - v/(c*c) - sqrt((o/(2*c) - v/(c*c)) * (o/(2*c) - v/(c*c)) * v/c))
Gleichung2 = Eq(b, o/(2*c) - v/(c*c) + sqrt((o/(2*c) - v/(c*c)) * (o/(2*c) - v/(c*c)) * v/c))
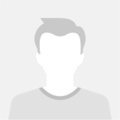
x=v/c
y=x/(2c)
z=O/(4c)
b=(z-y)+((z-y)**2-x)**0.5),(z-y)-((z-y)**2-x)**0.5)
Nicht getestet und ohne sonstige Garantien.
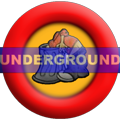
viele klammern und
import math
math.sqrt(x)
zum wurzel ziehen