Wie lautet der Code für den Punktestand in PingPong bei Python?
- Ping Pong Spiel in Python
- deutscher Code , für den Spielstand
- also wenn ein Tor fällt …
- der Spielstand soll angezeigt werden
1 Antwort
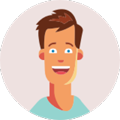
Frag doch mal ChatGPT, da kommt dann sowas bei raus:
Input:
Wie lautet der Code für den Punktestand in PingPong bei Python?
Ping Pong Spiel in Python
deutscher Code , für den Spielstand
also wenn ein Tor fällt …
der Spielstand soll angezeigt werden
Output:
Hier ist ein einfacher Python-Code für ein Ping-Pong-Spiel, der den Spielstand anzeigt, wenn ein Tor erzielt wird:
import pygame
import random
# Initialisierung von Pygame
pygame.init()
# Fenstergröße
WIDTH, HEIGHT = 800, 600
win = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Ping Pong Spiel")
# Farben
WHITE = (255, 255, 255)
# Schläger
player_width, player_height = 15, 100
player_x, player_y = 50, HEIGHT // 2 - player_height // 2
player_speed = 5
opponent_width, opponent_height = 15, 100
opponent_x, opponent_y = WIDTH - 50 - opponent_width, HEIGHT // 2 - opponent_height // 2
opponent_speed = 5
# Ball
ball_width, ball_height = 15, 15
ball_x, ball_y = WIDTH // 2 - ball_width // 2, HEIGHT // 2 - ball_height // 2
ball_speed_x = 5
ball_speed_y = random.choice([-5, 5])
# Punktestand
player_score = 0
opponent_score = 0
font = pygame.font.Font(None, 36)
# Spiel-Loop
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
keys = pygame.key.get_pressed()
if keys[pygame.K_w] and player_y > 0:
player_y -= player_speed
if keys[pygame.K_s] and player_y < HEIGHT - player_height:
player_y += player_speed
# Ball-Bewegung
ball_x += ball_speed_x
ball_y += ball_speed_y
# Ball-Kollision mit Wänden
if ball_y <= 0 or ball_y >= HEIGHT - ball_height:
ball_speed_y = -ball_speed_y
# Ball-Kollision mit Schlägern
if player_x + player_width >= ball_x >= player_x and player_y + player_height >= ball_y >= player_y:
ball_speed_x = -ball_speed_x
if opponent_x <= ball_x + ball_width <= opponent_x + opponent_width and opponent_y <= ball_y + ball_height <= opponent_y + opponent_height:
ball_speed_x = -ball_speed_x
# Ball aus dem Spielfeld
if ball_x <= 0:
opponent_score += 1
ball_x, ball_y = WIDTH // 2 - ball_width // 2, HEIGHT // 2 - ball_height // 2
if ball_x >= WIDTH:
player_score += 1
ball_x, ball_y = WIDTH // 2 - ball_width // 2, HEIGHT // 2 - ball_height // 2
# Zeichne alles
win.fill(WHITE)
player = pygame.draw.rect(win, WHITE, (player_x, player_y, player_width, player_height))
opponent = pygame.draw.rect(win, WHITE, (opponent_x, opponent_y, opponent_width, opponent_height))
ball = pygame.draw.ellipse(win, WHITE, (ball_x, ball_y, ball_width, ball_height))
player_text = font.render(f'Spieler: {player_score}', True, WHITE)
opponent_text = font.render(f'Gegner: {opponent_score}', True, WHITE)
win.blit(player_text, (20, 20))
win.blit(opponent_text, (WIDTH - opponent_text.get_width() - 20, 20))
pygame.display.update()
pygame.quit()
PS: Das ist ein komplettes Spiel
Woher ich das weiß:Recherche