Hallo,
ich erstelle gerade ein Flappy Bird Spiel, nur leider weiss ich nicht wie ich so ein Highscore + Score Anzeige bekomme:
Also ich glaube man braucht auch eine "Cloud-Variable" dafür und dazu möchte ich sagen, dass ich darauf Zugriff habe!
Wäre sehr nett, wenn mir jemand mir sagen könnte wie man sowas macht!
Das ist derzeitig mein Game Over Menü:
LG
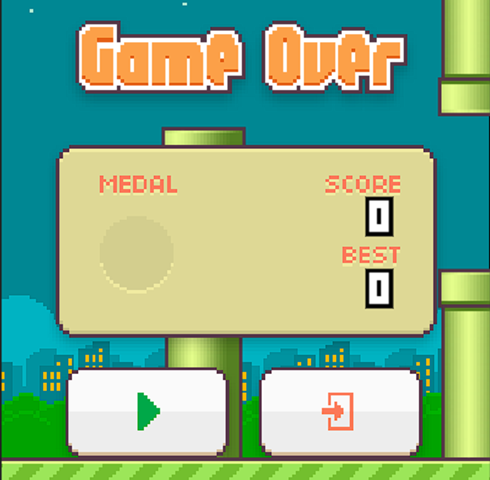