Wie Programm erstellen?
Ich suche für meine Arbeit eine Möglichkeit ein kleines Programm zu erstellen/schreiben.
Ich brauche dafür 14 Buttons (Bezeichnung A bis M). Im Grundzustand sind due Buttons grün. Sobald die A Taste auf der Tastertur gedrückt wird soll dieser Button (A Button) im sekundentakt, wie eine Art Alarm, abwechselt zwischen grün und roter Farbfüllung wechseln. So wie das H in meiner Skizze. Wird die gleiche Taste wieder gedrückt bleibt der Button wieder wie im Grundzustand grün. Dieses Programm soll permanent auf einem großen Bildschirm laufen. Was wäre die einfachste Möglichkeit sowas zu codieren?
2 Antworten
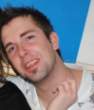
Ich hab mal ChatGPT bemüht, sowas zu bauen. Das kannst du einfach in einer HTML-Datei speichern und mit einem beliebigen Browser öffnen.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Blinking Buttons</title>
<style>
div {
display: flex;
flex-flow: row wrap;
}
.button {
display: inline-block;
padding: 10px 20px;
margin: 5px;
background-color: lightgreen;
border: 1px solid #ccc;
cursor: pointer;
flex: 1;
}
.blink {
animation: blink-animation 1s steps(2, jump-none) infinite;
}
@keyframes blink-animation {
to {
background-color: red;
}
}
</style>
</head>
<body>
<div id="buttons">
<button class="button" id="buttonA">A</button>
<button class="button" id="buttonB">B</button>
<button class="button" id="buttonC">C</button>
<button class="button" id="buttonD">D</button>
<button class="button" id="buttonE">E</button>
<button class="button" id="buttonF">F</button>
<button class="button" id="buttonG">G</button>
<button class="button" id="buttonH">H</button>
<button class="button" id="buttonI">I</button>
<button class="button" id="buttonJ">J</button>
<button class="button" id="buttonK">K</button>
<button class="button" id="buttonL">L</button>
<button class="button" id="buttonM">M</button>
<button class="button" id="buttonN">N</button>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function(){
$(document).keydown(function(event){
var keyPressed = String.fromCharCode(event.keyCode);
var buttonId = "#button" + keyPressed.toUpperCase();
$(buttonId).toggleClass("blink");
});
$("button").click(function(event){
$(event.target).toggleClass("blink");
})
});
</script>
</body>
</html>
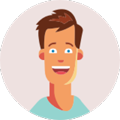
Am einfachsten sollte das als Webseite mit HTML, CSS und JavaScript für die Tastensteuerung und Farbänderung umsetzbar sein. Kann aber theoretisch auch mit jeder anderen Sprache / Technologie machen