Projekt Tigerjhyton?
Ich bin in der Schule und versuche ein Pong zu erstellen mit Tigerjhyton. Hätte mir jemand da eine Idee, die ich anschauen könnte?
2 Antworten
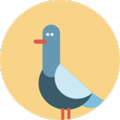
Von gutefrage auf Grund seines Wissens auf einem Fachgebiet ausgezeichneter Nutzer
Computer, Python
Ich habe vor langer Zeit mal Pong mit Pygame programmiert, da kannst du dir vielleicht paar Anregungen holen (ist nicht sehr gut, es lässt sich z.B. ziemlich einfach vorhersehen wo der Ball als nächstes landet):
import pygame
import random
import sys
from pygame.locals import (
K_UP,
K_DOWN,
K_LEFT,
K_RIGHT,
QUIT,
K_RETURN,
)
pygame.init()
pygame.font.init()
clock = pygame.time.Clock()
Black = (0, 0, 0)
Yellow = (255, 255, 0)
class Window():
def __init__(self):
self.WindowWide = 1000
self.WindowHight = 1000
self.WindowColour = (35, 35, 35)
Win = Window()
class Ball():
def __init__(self):
self.x = 500
self.y = 500
self.rad = 20
self.Colour = (0, 240, 0)
i = 1
while i < 2:
self.xspeed = random.randint(-5, 5)
self.yspeed = random.randint(-5, 5)
if self.xspeed < 3 and self.xspeed > -3:
continue
if self.yspeed < 3 and self.yspeed > -3:
continue
i = 2
def move(self):
if self.x >= (Win.WindowWide - self.rad):
self.xspeed = self.xspeed * (-1)
elif self.x <= (0 + self.rad):
self.xspeed = self.xspeed * (-1)
elif self.y <= (0 + self.rad):
self.yspeed = self.yspeed * (-1)
self.y += self.yspeed
self.x += self.xspeed
class Paddle():
def __init__(self):
self.x = 400
self.y = 950
self.speed = 5
self.wide = 100
self.hight = 15
self.PaddleColour = (240, 0, 0)
# Inizialisieren
win = pygame.display.set_mode((Win.WindowWide, Win.WindowHight))
ball = Ball()
pad = Paddle()
ball2 = Ball()
MainRunning = True
while MainRunning:
buttons = [True, False, False, False]
buttonNumber = 0
ball.Colour = (0, 255, 0)
ball2.Colour = (0, 255, 0)
running1 = True
while running1:
pressed_keys = pygame.key.get_pressed()
for event in pygame.event.get():
if event.type == QUIT:
running1 = False
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == K_UP:
if buttonNumber > 1:
buttonNumber -= 2
else:
buttonNumber += 2
elif event.key == K_DOWN:
if buttonNumber < 2:
buttonNumber += 2
else:
buttonNumber -= 2
elif event.key == K_RIGHT:
if buttonNumber == 3:
buttonNumber -= 3
else:
buttonNumber += 1
elif event.key == K_LEFT:
if buttonNumber == 0:
buttonNumber += 3
else:
buttonNumber -= 1
elif event.key == K_RETURN:
ball.x = random.randint(490, 510)
ball.y = random.randint(490, 510)
ball2.x = random.randint(490, 510)
ball2.y = random.randint(490, 510)
running1 = False
if pressed_keys[pygame.K_ESCAPE]:
running1 = False
pygame.display.flip()
win.fill((80, 80, 80))
pygame.draw.circle(win, (130, 230, 130), (ball.x, ball.y), ball.rad)
pygame.draw.rect(win, (230, 120, 120), (pad.x, pad.y, pad.wide, pad.hight))
clock.tick(60)
running2 = True
while running2:
ballframe = pygame.Rect((ball.x - ball.rad), (ball.y - ball.rad), (ball.rad * 2), (ball.rad * 2))
if buttons[2] or buttons[3]:
ballframe2 = pygame.Rect(ball2.x, ball2.y, ball2.rad, ball2.rad)
ballframeleft = pygame.Rect((ball2.x + ball2.rad - 2), (ball2.y - ball2.rad), 2, (ball2.rad * 2))
ballframeright = pygame.Rect((ball2.x - ball2.rad), (ball2.y + ball2.rad), 2, (ball2.rad * 2))
ballframeup = pygame.Rect((ball2.x - ball2.rad), (ball2.y - ball2.rad), (ball2.rad * 2), 2)
ballframedown = pygame.Rect((ball2.x - ball2.rad), (ball2.y + ball2.rad - 2), (ball2.rad * 2), 2)
padframe = pygame.Rect(pad.x, pad.y, pad.wide, pad.hight)
pressed_keys = pygame.key.get_pressed()
for event in pygame.event.get():
if event.type == QUIT:
running2 = False
sys.exit()
if pressed_keys[pygame.K_ESCAPE]:
running2 = False
if pressed_keys[pygame.K_RIGHT] and pad.x < (1000 - pad.wide):
pad.x += 7
elif pressed_keys[pygame.K_LEFT] and pad.x > 0:
pad.x += -7
pygame.display.flip()
win.fill(Win.WindowColour)
pygame.draw.circle(win, ball.Colour, (ball.x, ball.y), ball.rad)
if buttons[2] or buttons[3]:
pygame.draw.circle(win, ball2.Colour, (ball2.x, ball2.y), ball2.rad)
pygame.draw.rect(win, pad.PaddleColour, (pad.x, pad.y, pad.wide, pad.hight))
if ballframe.colliderect(padframe):
ball.yspeed *= (-1)
ball.move()
if ball.y >= (Win.WindowHight - ball.rad):
ball.yspeed = ball.yspeed
ball.Colour = (250, 0, 10)
running2 = False
if buttons[2] or buttons[3]:
if ballframe2.colliderect(padframe):
ball2.yspeed *= (-1)
ball2.move()
clock.tick(60)
Woher ich das weiß:Hobby – Programmiere seit eineinhalb Jahren
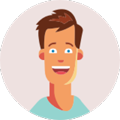
Von gutefrage auf Grund seines Wissens auf einem Fachgebiet ausgezeichneter Nutzer
programmieren, Python
Hier mal mein TicTacToe in Python >=3.5
Du spielst einen Zug, und dann der Computer. Der Computer ist aber nicht besonders schlau ;)
Mit boardSize kannst du auch ein n*n großes Feld einstellen.
import random
def checkBoard(board, boardSize):
#checking if any row won
for row in board:
checkRows = True
fPiece = row[0]
for i in row:
if fPiece != 0:
if i != fPiece:
checkRows = False
break
else:
checkRows = False
if checkRows: break
#checking if any column won
for j in range(boardSize):
checkCols = True
fPiece = board[0][j]
for i in range(boardSize):
if fPiece != 0:
if fPiece != board[i][j]:
checkCols = False
break
else:
checkCols = False
if checkCols: break
#checking if diagonal right upper corner to left lower corner won
checkDia = True
fPiece = board[0][-1]
for i in range(boardSize):
if fPiece != 0:
if board[i][boardSize-(i+1)] != fPiece:
checkDia = False
break
else:
checkDia = False
if not checkDia:
#checking second diagonal if first on didn't allready win
checkDia = True
fPiece = board[0][0]
for i in range(boardSize):
if fPiece != 0:
if board[i][i] != fPiece:
checkDia = False
break
else:
checkDia = False
return ((checkRows or checkCols or checkDia), fPiece)
def nextMove(board, boardSize):
boardCopy = board.copy()
winningMove = False
for i in range(boardSize):
for j in range(boardSize):
if boardCopy[i][j] == 0:
boardCopy[i][j] = 2
winningMove,pid = checkBoard(boardCopy, boardSize)
if winningMove:
x,y = j,i
break
boardCopy[i][j] = 0
if winningMove:
break
if winningMove:
return (x,y)
loosingMove = False
for i in range(boardSize):
for j in range(boardSize):
if boardCopy[i][j] == 0:
boardCopy[i][j] = 1
loosingMove,pid = checkBoard(boardCopy, boardSize)
if loosingMove:
x,y = j,i
break
boardCopy[i][j] = 0
if loosingMove:
break
if loosingMove:
return (x,y)
if boardCopy[boardSize//2][boardSize//2] == 0:
return (boardSize//2,boardSize//2)
possibleMoves = []
for i in range(boardSize):
for j in range(boardSize):
if board[i][j] == 0:
possibleMoves += [[j,i]]
return random.choice(possibleMoves)
def printBoard(board, boardSize, symbols):
print(' '+' '.join(str(n) for n in range(boardSize)))
for line in range(len(board)):
s = '|'.join(symbols[i] for i in board[line])
space = ' '*(len(str(boardSize))-len(str(line))+1)
print(f'{line}{space}{s}')
boardSize = 3
board = [[0 for j in range(boardSize)] for i in range(boardSize)]
won = False
boardFull = False
symbols = ['#','O','X'] #symbols 0=>emtpy,1=> player1 piece, 2=>player2 piece
playerTurn = 0
printBoard(board, boardSize, symbols)
movesCounter = 0
maxMoves = boardSize*boardSize
while not won and not boardFull:
if playerTurn == 0:
#get user input
inputOk = False
while not inputOk:
#aks for input until input is in x,y format
#get input and remove spaces
moveChoice = input(f'Player {playerTurn+1} please pick your move[x,y]: ').replace(' ','')
try:
x,y = moveChoice.split(',') #trying to split x,y at ,
x = int(x)
y = int(y)
if (0 <= x <= boardSize-1) and (0 <= y <= boardSize-1) and (board[y][x]==0):
#checking if x and y between boardSize and board Position is empty
inputOk = True
else:
#either x or y to large or board not empty at position
print('Please only input allowed Values!')
except ValueError:
#either x or y not int or no not able to split input
print('Please only input allowed Values!')
else:
x,y = nextMove(board, boardSize)
print('Computer has played')
board[y][x] = playerTurn+1 #laying piece on board
won,pid = checkBoard(board, boardSize)
movesCounter += 1 #counting pieces on board
printBoard(board, boardSize, symbols) #printing board
playerTurn = not playerTurn #setting player from 0=>1 or 1=>0
#checking if board is full
if movesCounter == maxMoves and not won:
print('Tie!')
boardFull = True
if won:
print(f'Player {pid} has won the game!')
Woher ich das weiß:Studium / Ausbildung – Informatikstudent