Kann Javascript und/oder Python auch mit mySQL?
Kann ich mit einer oder beiden der genannten Sprachen auch mit Datenbanken wie mySQL arbeiten? Zum Beispiel ein Web-Lexikon schreiben oder ein Backend für Webseiten erstellen? Oder ist der "Klassiker" PHP absoluter Platzhirsch in dem Bereich?
2 Antworten
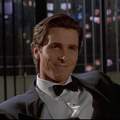
Sowohl JavaScript als auch Python können auch als Backend betrieben werden. Bei JavaScript geht das mit einer entsprechenden Server-Runtime wie Node.js, Deno oder Bun (Node.js ist dabei immer noch Marktführer, auch wenn Deno inzwischen gut aufholt). Python kann theoretisch auch "vanilla", d.h. oder zusätzliche Runtimes und Frameworks, auf dem Server betrieben werden (einfach Python auf dem Server installieren...), aber einfacher macht man es sich mit etwas wie Django oder Flask.
PHP ist absolut nicht mehr "Platzhirsch" im Backend-Bereich (Gott sei Dank).
Der einzige Nachteil den man hat ist, dass auf Shared Webhosts, die im Cent- bis Eurobereich verramscht werden, oft nur PHP-Applikationen installiert werden können. Will man Node oder gar Python, muss man oft entweder auf einen teureren Webhost zurück greifen, der das erlaubt (oft aber trotzdem mit Einschränkungen), oder aber entweder einen eigenen Server betreiben oder einen PaaS (Platform as a Service) Dienst wie Heroku nutzen.
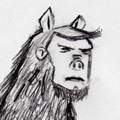
Ja das geht definitiv. Es ist ein klassischer Anwendungsfall.
Das wird in der Webentwicklung im Backend gemacht. PHP wird immer mehr abgelöst von JavaScript und Python, da es ein eher veraltetes und nicht so mächtiges Ökosystem hat.
Im Frontend läuft nach wie vor HTML, CSS und JavaScript. Im Backend dann PHP, Python oder auch JavaScript um Anfragen des Frontends entgegenzunehmen und mit Datenbanken interagiert.
Beispiel-Code (Python):
import mysql.connector
# Connect to the database
cnx = mysql.connector.connect(user='your_username', password='your_password', host='your_host', database='your_database')
# Create a cursor to execute the SELECT statement
cursor = cnx.cursor()
# Execute the SELECT statement
cursor.execute("SELECT * FROM example_table")
# Fetch all the rows of the result
rows = cursor.fetchall()
# Store the result in a list
result_list = [list(row) for row in rows]
# Close the cursor and connection
cursor.close()
cnx.close()
print(result_list)
JavaScript:
const mysql = require('mysql2');
// Create a connection to the database
const connection = mysql.createConnection({
host: 'your_host',
user: 'your_username',
password: 'your_password',
database: 'your_database'
});
// Connect to the database
connection.connect(function(err) {
if (err) throw err;
// Execute the SELECT statement
connection.query('SELECT * FROM example_table', function (error, results) {
if (error) throw error;
// Store the result in a variable
const resultList = results;
// Close the connection
connection.end();
console.log(resultList);
});
});
Wichtig: Dieser Code muss serverseitig laufen (im Backend).